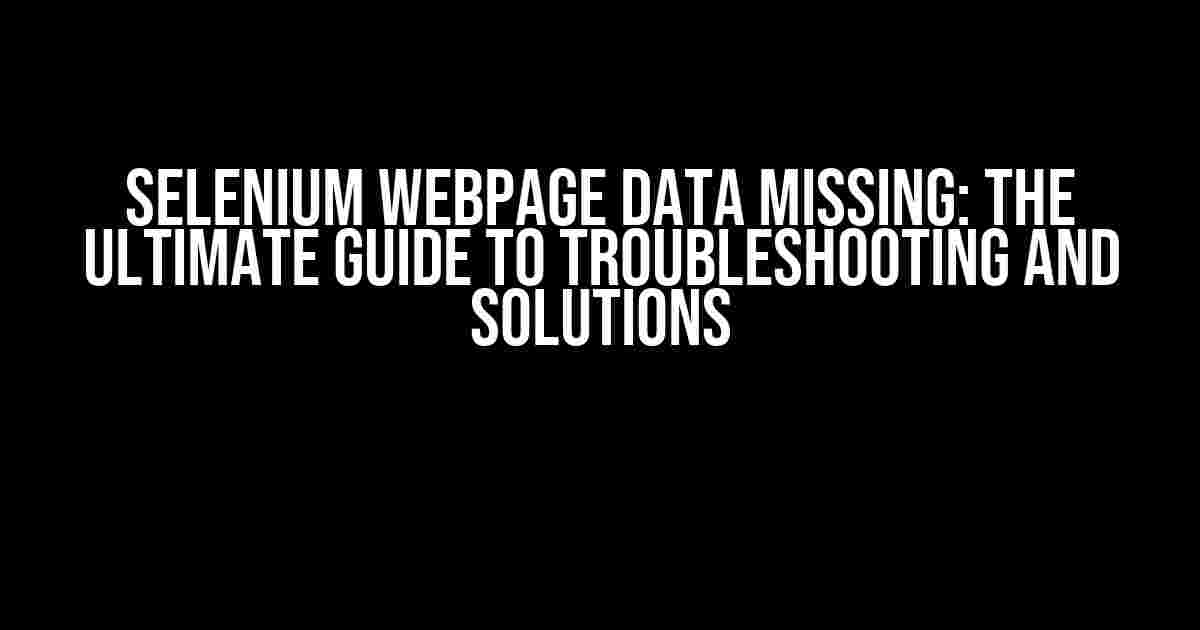
Selenium Webpage Data Missing: The Ultimate Guide to Troubleshooting and Solutions
Posted onAre you frustrated with Selenium webpage data missing issues? Do you spend hours debugging and still can’t find the solution? You’re not alone! In this article, we’ll dive into the common reasons behind Selenium webpage data missing, troubleshoot common issues, and provide you with actionable solutions to get your data back.
Understanding Selenium Webpage Data Missing
Selenium is an incredible tool for automating web browsing and scraping data. However, sometimes, it can be frustrating when Selenium webpage data goes missing. But what exactly happens when data goes missing?
Selenium uses a web driver to interact with web pages. When you navigate to a webpage, Selenium loads the HTML content and extracts the data you need. However, if the data is not present in the HTML or is loaded dynamically, Selenium might not be able to find it. This results in Selenium webpage data missing.
Common Reasons for Selenium Webpage Data Missing
- Dynamically Loaded Content: Some web pages load content dynamically using JavaScript, making it difficult for Selenium to extract data.
- AJAX and XHR Requests: AJAX and XHR requests can load data in the background, making it challenging for Selenium to capture the data.
- Loading Times: Slow-loading web pages can cause Selenium to time out before the data is fully loaded.
- Anti-Scraping Measures: Some websites employ anti-scraping measures, such as CAPTCHAs, to prevent bots from extracting data.
- JavaScript-Heavy Pages: Pages with heavy JavaScript usage can cause Selenium to struggle with extracting data.
Troubleshooting Selenium Webpage Data Missing
Before we dive into solutions, let’s troubleshoot the most common issues causing Selenium webpage data missing.
1. Checking the HTML Source
First, inspect the HTML source code of the webpage using the browser’s developer tools. Check if the data you’re looking for is present in the HTML.
<html> <head></head> <body> <p>This is the data I'm looking for</p> </body> </html>
If the data is not present in the HTML, move on to the next step.
2. Checking for Dynamic Content
Use Selenium’s built-in `execute_script` method to execute a JavaScript command that waits for the dynamic content to load.
driver.execute_script("return document.readyState").equals("complete")
This code waits for the page to finish loading and ensures that the dynamic content is present.
3. Inspecting Network Requests
Use the browser’s developer tools to inspect the network requests made by the webpage. Check for any XHR or AJAX requests that might be loading the data you need.
Right-click on the request and select “Copy as cURL” to get the request details.
curl 'https://example.com/data' -H 'User-Agent: Mozilla/5.0' -H 'Accept: application/json'
Use this information to create a custom request using Selenium’s `requests` library.
4. Handling Anti-Scraping Measures
Identify the anti-scraping measures employed by the website, such as CAPTCHAs. Use a CAPTCHA-solving service or implement a custom solution to handle these challenges.
Solutions for Selenium Webpage Data Missing
Now that we’ve troubleshooted the common issues, let’s explore solutions to get your data back.
1. Using Selenium’s WebDriverWait
Use Selenium’s `WebDriverWait` class to wait for the element containing the data to be present.
from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC element = WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.ID, "myElement")) )
This code waits for up to 10 seconds for the element with the ID “myElement” to be present before throwing a timeout exception.
2. Using Selenium’s execute_script Method
Use Selenium’s `execute_script` method to execute a JavaScript command that extracts the data.
data = driver.execute_script("return document.getElementById('myElement').textContent")
This code executes a JavaScript command that returns the text content of the element with the ID “myElement”.
3. Using a Custom Request with Requests Library
Use the `requests` library to create a custom request that loads the data.
import requests url = "https://example.com/data" headers = {"User-Agent": "Mozilla/5.0", "Accept": "application/json"} response = requests.get(url, headers=headers) data = response.json()
This code sends a GET request to the specified URL with the headers and retrieves the JSON data.
4. Using a CAPTCHA-Solving Service
Use a CAPTCHA-solving service, such as 2Captcha or DeathByCaptcha, to handle anti-scraping measures.
import requests api_key = "YOUR_API_KEY" captcha_url = "https://example.com/captcha" response = requests.post( f"http://2captcha.com/in.php?key={api_key}&method=userrecaptcha&googlekey=6Le-wvkSAAAAAPBM8p2rQ McLdK", json={"url": captcha_url}, ) captcha_id = response.json()["request"] response = requests.get(f"http://2captcha.com/res.php?key={api_key}&action=get&id={captcha_id}") captcha_solution = response.json()["request"]
This code uses the 2Captcha API to solve a CAPTCHA challenge.
Best Practices for Selenium Webpage Data Extraction
To avoid Selenium webpage data missing issues, follow these best practices:
- Inspect the HTML Source: Always inspect the HTML source code to ensure the data is present.
- Use WebDriverWait: Use Selenium’s `WebDriverWait` class to wait for elements to be present.
- Avoid Over-Engineering: Keep your code simple and avoid over-engineering solutions.
- Test Thoroughly: Test your code thoroughly to ensure it works as expected.
- Respect Website Terms: Always respect website terms and conditions, and avoid scraping data that’s not intended for public use.
Conclusion
Selenium webpage data missing can be frustrating, but with the right troubleshooting techniques and solutions, you can overcome these challenges. By following the best practices and using the solutions outlined in this article, you’ll be well on your way to extracting data efficiently and effectively.
Solution | Description |
---|---|
Using Selenium’s WebDriverWait | Wait for elements to be present before extracting data |
Using Selenium’s execute_script Method | Execute a JavaScript command to extract data |
Using a Custom Request with Requests Library | Send a custom request to load data |
Using a CAPTCHA-Solving Service | Solve CAPTCHA challenges to access data |
Remember, scraping data from websites should always be done with caution and respect for website terms and conditions.
Here are the 5 Questions and Answers about “Selenium webpage data missing” in a creative voice and tone:
Frequently Asked Questions
If you’re struggling to extract data from a webpage using Selenium, you’re not alone! We’ve got the answers to some of the most common questions about missing webpage data.
Why is Selenium not able to find the webpage elements I want to scrape?
This might be because the elements are loaded dynamically by JavaScript. Try using WebDriverWait to wait for the elements to load before trying to scrape them. You can also try using a headless browser like PhantomJS or HtmlUnit, which can load JavaScript-heavy pages more efficiently.
What if the webpage uses a lot of AJAX calls to load its data?
Selenium can struggle with AJAX-heavy pages because it can’t detect when all the data has finished loading. Try using Selenium’s built-in waiting mechanisms, like WebDriverWait, to wait for specific elements to appear on the page. You can also use tools like Selenium WebDriver’s FluentWait to wait for the page to stabilize before scraping.
Why do I get a StaleElementReferenceException when trying to scrape a webpage?
This error usually occurs when the webpage has already changed or reloaded by the time Selenium tries to interact with it. Try using a more specific and unique locator to identify the element you want to scrape, and make sure you’re waiting for the page to finish loading before attempting to scrape.
How can I handle pop-up windows or alerts that block Selenium from scraping the webpage?
Selenium provides several ways to handle alerts and pop-ups. You can use the Alert class to dismiss or accept alerts, or use the SwitchTo method to switch between windows. For more complex scenarios, you can use a library like PyQt or PyAutoGUI to automate interactions with the pop-up window.
What if the webpage uses anti-scraping measures, like CAPTCHAs or rate limiting?
Anti-scraping measures can be tough to overcome. Try using a more advanced scraping library like Scrapy, which has built-in support for handling CAPTCHAs and rate limiting. You can also use services like 2Captcha or DeathByCaptcha to solve CAPTCHAs programmatically. Just remember to always respect the website’s terms of service and robots.txt file!